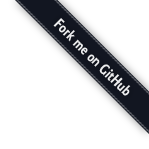
Opening a Dialog
view codeOpening a static Dialog
The layout of the Dialog is fully described by the referenced HTML template: either the default "#dialog_generic" of a specific one.
You can fully customize the rendering with CSS; the default styles are provided by static/frontend_forms/css/frontend_forms.css
dialog1 = new Dialog({ dialog_selector: '#dialog_generic', html: '<h1>Static content goes here ...</h1>', width: '600px', min_height: '200px', title: '<i class="fa fa-calculator"></i> Select an object ...', footer_text: 'testing dialog ...', enable_trace: true }); dialog1.open()
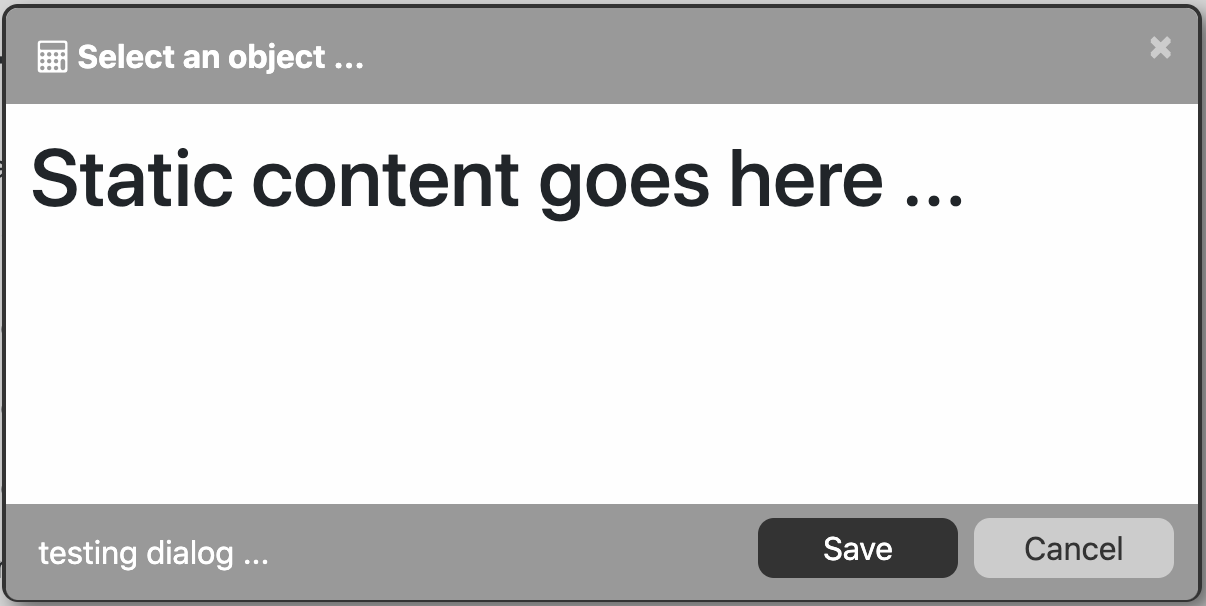
A simple static Dialog
Opening a dynamic Dialog
In most cases, you will rather produce the dialog content dynamically.
To obtain that, just add an "url" option to the Djalog constructor, and it will be automatically used to obtain the Dialog content from the server via an Ajax call.
dialog1 = new Dialog({ ... url: "/samples/simple-content", ...
Sometimes it is convenient to reuse the very same single view to render either a modal dialog, or a standalone HTML page.
This can be easily accomplished providing:
- an "inner" template which renders the content
- an "outer" container template which renders the full page, then includes the "inner" template
- in the view, detect the call context and render one or another
def simple_content2(request): # Either render only the modal content, or a full standalone page if request.is_ajax(): template_name = 'frontend/includes/simple_content2_inner.html' else: template_name = 'frontend/includes/simple_content2.html' return render(request, template_name, { })
here, the "inner" template provides the content:
<div class="row"> <div class="col-sm-4"> <p>Quam aliquam possimus? Ab illo facere expedita atque velit quisquam ex quibusdam cum, deleniti accusantium earum numquam, et eos neque, facilis harum aliquid tempora perspiciatis sint illo tenetur incidunt, reiciendis quasi harum veritatis asperiores maiores facere totam expedita magni. Impedit voluptatem dolorum qui repudiandae provident sunt fugit, voluptatum velit odio deserunt facilis doloribus nihil, nobis nisi quo quod iure vel atque in tempora illo debitis, architecto nihil mollitia rem nostrum molestias similique? Amet ipsum perferendis ipsa minus dolorum omnis enim, nihil libero impedit sed reiciendis minima nostrum iste minus eligendi ut nemo, quaerat laudantium tempora libero magnam quisquam debitis iure architecto accusamus atque.</p> </div> <div class="col-sm-4"> <p>Inventore eum beatae ea eligendi dolor sed et, maiores officia nesciunt qui facilis cum molestias sapiente voluptatem recusandae atque, suscipit aspernatur quisquam, natus distinctio quae ab libero quidem asperiores vitae dolorem voluptatibus, architecto deserunt modi blanditiis eos veritatis? Facilis expedita officiis omnis, deleniti quasi ipsum quaerat ipsa?</p> </div> <div class="col-sm-4"> <p>Ullam temporibus quam vitae ea, doloribus deserunt commodi omnis dicta expedita vero, tempora eius officiis itaque perspiciatis perferendis expedita illum dignissimos odio, nostrum consectetur unde quo commodi ea maiores, est corporis corrupti eligendi architecto ex expedita quia accusamus ipsa. Error iste ea placeat ratione distinctio? Dolorem quo ipsam iste voluptate sapiente expedita sunt aspernatur modi, officiis sed quis ratione debitis doloremque mollitia quam perferendis odio deleniti. Nihil necessitatibus minima molestias quidem delectus voluptate, repellendus totam rem numquam.</p> </div> </div>
while the "outer" one renders the full page:
{% extends "base.html" %} {% load static staticfiles i18n %} {% block content %} {% include 'frontend/includes/simple_content2_inner.html' %} {% endblock content %}